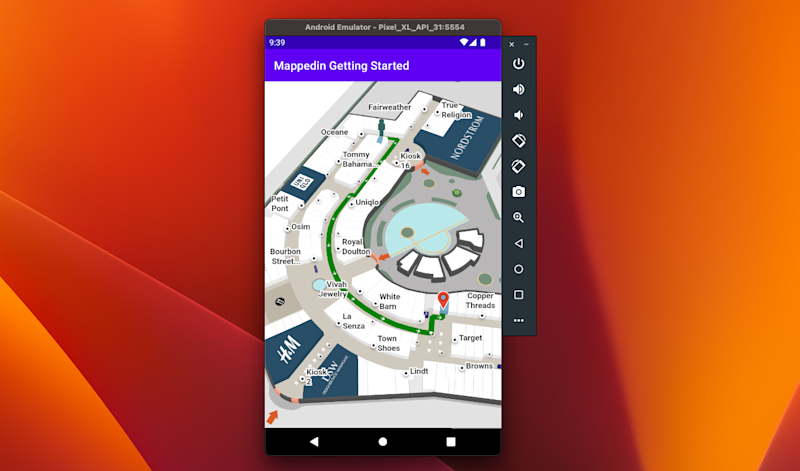
Wayfinding is one of the most often used features of digital maps and the Mappedin Android SDK makes it incredibly easy to draw a path from A to B. It only takes a known start and end location to generate directions, which can be passed to MPIJourneyManager
to draw it on the map.
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: ABWayfinding.kt
Single-Destination Wayfinding
We can use the onFirstMapLoaded
function of the MPIMapViewListener
to present wayfinding directions when the map loads. Select your departure and destination locations from venueData
and supply them as the "to" and "from" for getDirections()
. In the callback, pass the resulting directions to journeyManager.draw()
to display the route.
override fun onFirstMapLoaded() { val departure = mapView.venueData?.locations?.first { it.name == "Pet World" }!! val destination = mapView.venueData?.locations?.first { it.name == "Microsoft" }!!
mapView.getDirections(to = destination, from = departure) { if (it != null) { mapView.journeyManager.draw(directions = it) } }}
journeyManager
exposes the MPIJourneyManager
helper class to display single and multi-destination wayfinding easily on the map. Functionality of the Journey
could be replicated by drawing the paths and adding well designed tooltips at connection points.
Wayfinding From A User's Location
To find out how to start the journey from the user's current location, refer to Wayfinding from Blue Dot in the Blue Dot Guide.
Multi-Floor Wayfinding
Using MPIJourneyManager
helper, no additional work is needed to provide wayfinding between floors. Whenever a user needs to switch a floor, an interactive tooltip with an icon indicating the type of connection (such as an elevator or escalator) will be drawn. By tapping the tooltip, the map view switches to the destination floor.
Wayfinding Using Accessible Routes
When requesting directions for a journey, it is important to consider the needs of the user. Users with mobility restrictions may require a route that avoids stairs and escalators and instead uses ramps or elevators.
The MPIMapView.getDirections() method accepts an accessible boolean flag, which allows specifying whether an accessible route should be returned. By default, the shortest available route is chosen. The following code demonstrates how to request directions that make use of accessible routes.
mapView.getDirections(to = destination, from = departure, accessible = true) { if (it != null) { mapView.journeyManager.draw(directions = it) }}
Multi-Destination Wayfinding
With the Mappedin SDK, it's possible to draw a path including multiple waypoints. To generate directions, create an MPIDestinationSet
from polygons, locations or nodes and request directions from a starting location with getDirections()
.
Create a couple properties for the UIViewController which we will use to store the current step number and the destination list. Use the onFirstMapLoaded
function in MPIMapViewListener
to draw the route once the map is ready.
private var step = 0private var destinations = emptyList<MPINavigatable.MPILocation?>()
override fun onFirstMapLoaded() { val departure = mapView.venueData?.locations?.first { it.name == "Parking Lot E" }!! destinations = listOf( mapView.venueData?.locations?.first { it.name == "Apple" }, mapView.venueData?.locations?.first { it.name == "Microsoft" }, mapView.venueData?.locations?.first { it.name == "ThinkKitchen" }, mapView.venueData?.locations?.first { it.name == "Parking Lot E" } ) mapView.getDirections(to = MPIDestinationSet(destinations = destinations as List<MPINavigatable>), from = departure) { if (it != null) { mapView.journeyManager.draw(directions = it) } }}
Once the user has completed a segment of the journey, the active step can be changed with journeyManager.setStep()
. The following will move through the steps when user clicks outside of interactive polygons on the map using the onNothingClicked
function.
override fun onNothingClicked() { step += 1 mapView.journeyManager.setStep(step = step % destinations.size) { it?.message?.let { Log.e("MPIMapViewListener", it) } }}
Wayfinding Without Using Journey
While Journey
is a great utility for drawing a single path with all the features, it may sometimes be limiting. For instance, you may only draw one Journey
at a time, and subsequent calls to journeyManager.draw()
will overwrite the previous.
For full control over the route, draw the path using the MPIPathManager.add()
method within the MPIMapView
and pass it the directions.path
.
override fun onFirstMapLoaded() { val departure = mapView.venueData?.locations?.first { it.name == "ThinkKitchen" }!! val destination = mapView.venueData?.locations?.first { it.name == "American Eagle" }!!
mapView.getDirections(to = destination, from = departure) { it?.let { mapView.pathManager.add(path = it.path) } }}
This creates a basic path with no extra features. Using this method, you will need to handle both multi-floor navigation and multi-destination wayfinding manually using markers and by drawing multiple paths.
We can draw a secondary path the same way as the first while providing different start and end locations. To make this new path stand out, style it a different color by changing the color
property. The documentation for MPIOptions.Path covers the other options available to you.
mapView.pathManager.add(path = it.path, MPIOptions.Path(color = "green"))
X-Ray Paths
X-Ray Paths are currently an experimental component of the Mappedin SDK. While in this state, some features may not behave as intended and APIs may be changed. Experimental features are being tested together with early adopters to fine-tune them.
Maps with narrow corridors can benefit from using X-Ray Paths for wayfinding. When enabled, X-Ray Paths cause polygons, such as walls or desks, to become transparent when a path is drawn behind them. This allows the path to remain visible. The screenshot below illustrates a path between cabins on a cruise ship with X-Ray Paths disabled on the left and enabled on the right.
X-Ray Paths are enabled and disabled using the boolean xRayPath
parameter of MPIOptions.ShowVenue object passed to loadVenue.
X-Ray Paths are enabled by default, but they do require multiBufferRendering
to also be enabled. multiBufferRendering
is currently disabled by default. The code sample below demonstrates enabling both xRayPath
and multiBufferRendering
.
mapView.loadVenue( MPIOptions.Init( "clientID", "clientSecret", "venueSlug", ), MPIOptions.ShowVenue(multiBufferRendering = true, xRayPath = true),) { Log.e(javaClass.simpleName, "Error loading map view") }
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: ABWayfinding.kt