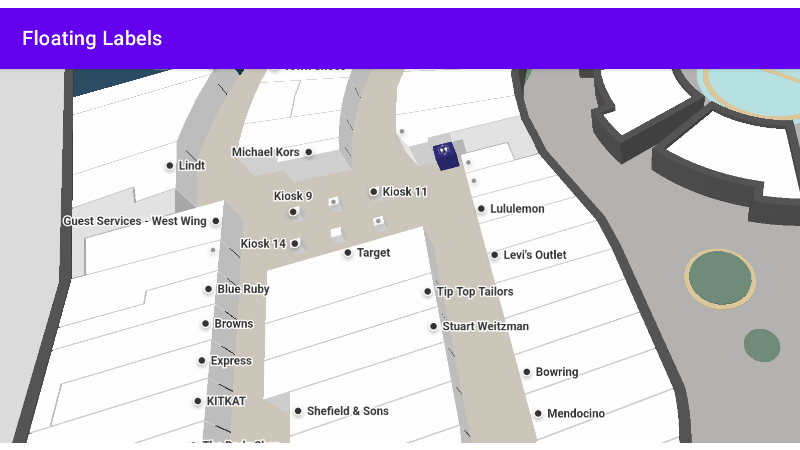
Map geometry alone is not enough to have a sense of a location. Adding labels to show the names of locations and other points of interest can help users navigate a space.
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: FloatingLabels.kt
FloatingLabels anchor to polygon entrance nodes and automatically handle rotation and repositioning when the camera moves. Floating Labels are enabled by default with default styling. When customizing Floating Labels, disable them from appearing on startup in ShowVenue
options. Floating Labels can be added to the map by calling mapView.floatingLabelsManager.labelAllLocations()
. This call should be made after MPIMapViewListener.onFirstMapLoaded is called, which indicates that the first map is loaded and can be modified.
Floating Labels can be added all at once as shown above or individually using the MPIFloatingLabelsManager.add() and MPIFloatingLabelsManager.remove() methods.
Styling Options
Floating Labels are highly customizable, allowing control of size, colour, spacing and the ability to display both a Marker (icon) and text in each label.
margin
in pixels around the label and marker. This will affect label density.
Marker Appearance - MPIOptions.FloatingLabelAppearance.Marker
backgroundColor
&foregroundColor
- The marker takes both active and inactive variants of its foreground and background colors.size
of the marker in pixels.icon
is the SVG of the icon to place inside the Floating Label.iconVisibilityThreshold
defines when the icon becomes visible relative to the current zoom level. Values should be between 0 and 1. 0 appears at maximum zoom and 1 causes the marker to always be shown.
Text Appearance - MPIOptions.FloatingLabelAppearance.Text
foregroundColor
andbackgroundColor
can be set using CSS colors, as Hex strings or the CSS name of a color such as crimson.maxWidth
defines the maximum width of text in pixels.numLines
sets the maximum number of lines to display if the text spans multiple lines.size
is the text size in pixels.
Adding Icons
Icons can be added to Floating Labels to highlight certain categories or to make locations more eye-catching. These icons automatically adjust, becoming visible as the user zooms the map to view more detail. The supplied icon must be an SVG image and is provided to MPIOptions.FloatingLabelAppearance.Marker
. Note that the SVG must be encapsulated using triple double quotes (“””SVG”””) to avoid double escaping characters within the string.
val svgIcon: String = """ <svg width="92" height="92" viewBox="-17 0 92 92" fill="none" xmlns="http://www.w3.org/2000/svg"> <g clip-path="url(#clip0)"> <path d="M53.99 28.0973H44.3274C41.8873 28.0973 40.7161 29.1789 40.7161 31.5387V61.1837L21.0491 30.7029C19.6827 28.5889 18.8042 28.1956 16.0714 28.0973H6.5551C4.01742 28.0973 2.84619 29.1789 2.84619 31.5387V87.8299C2.84619 90.1897 4.01742 91.2712 6.5551 91.2712H16.2178C18.7554 91.2712 19.9267 90.1897 19.9267 87.8299V58.3323L39.6912 88.6656C41.1553 90.878 41.9361 91.2712 44.669 91.2712H54.0388C56.5765 91.2712 57.7477 90.1897 57.7477 87.8299V31.5387C57.6501 29.1789 56.4789 28.0973 53.99 28.0973Z" fill="white"/> <path d="M11.3863 21.7061C17.2618 21.7061 22.025 16.9078 22.025 10.9887C22.025 5.06961 17.2618 0.27124 11.3863 0.27124C5.51067 0.27124 0.747559 5.06961 0.747559 10.9887C0.747559 16.9078 5.51067 21.7061 11.3863 21.7061Z" fill="white"/> </g> <defs> <clipPath id="clip0"> <rect width="57" height="91" fill="white" transform="translate(0.747559 0.27124)"/> </clipPath> </defs> </svg>""".trimIndent()
// Define colors using RGB values.val foreGroundColor: MPIOptions.FloatingLabelAppearance.Color = MPIOptions.FloatingLabelAppearance.Color(active = "#BF4320", inactive = "#7E2D16")val backgroundColor: MPIOptions.FloatingLabelAppearance.Color = MPIOptions.FloatingLabelAppearance.Color(active = "#FFFFFF", inactive = "#FAFAFA")
val markerAppearance: MPIOptions.FloatingLabelAppearance.Marker = MPIOptions.FloatingLabelAppearance.Marker( foregroundColor = foreGroundColor, backgroundColor = backgroundColor, icon = svgIcon,)
val markerTheme: MPIOptions.FloatingLabelAppearance = MPIOptions.FloatingLabelAppearance(marker = markerAppearance)val themeOptions: MPIOptions.FloatingLabelAllLocations = MPIOptions.FloatingLabelAllLocations(markerTheme)
mapView.floatingLabelsManager.removeAll()mapView.floatingLabelsManager.labelAllLocations(themeOptions)
Preset Themes
The Mappedin SDK for Android provides two preset Floating Label themes that can be easily applied to a map. They are lightOnDark
and darkOnLight
. These two contrasting themes provide options for both light and dark maps.
Applying the lightOnDark Theme
val themeOptions: MPIOptions.FloatingLabelAllLocations = MPIOptions.FloatingLabelAllLocations(MPIOptions.FloatingLabelAppearance.lightOnDark)mapView.floatingLabelsManager.removeAll()mapView.floatingLabelsManager.labelAllLocations(themeOptions)
Applying the darkOnLight Theme
val themeOptions: MPIOptions.FloatingLabelAllLocations = MPIOptions.FloatingLabelAllLocations(MPIOptions.FloatingLabelAppearance.darkOnLight)mapView.floatingLabelsManager.removeAll()mapView.floatingLabelsManager.labelAllLocations(themeOptions)
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: FloatingLabels.kt