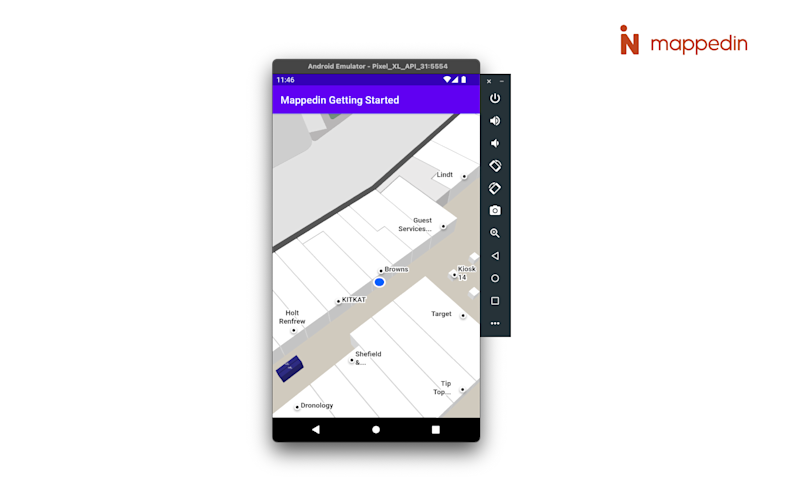
Displaying user position inside a building is not accurate with just GPS. However, there are a multitude of Indoor Positioning Systems utilizing different technological approaches to providing an accurate and reliable positioning inside. Mappedin works with any IPS capable of providing the SDK with latitude, longitude and floor level information.
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: BlueDot.kt
Enabling Blue Dot
You can initialize and enable the Blue Dot when the map first loads by using the onFirstMapLoaded
-function implemented by MPIMapViewDelegate
. The MPIBlueDotManager
can be easily enabled with default settings by calling mapView.blueDotManager.enable(MPIOptions.BlueDot())
.
There are two useful events to listen to: position update and state change. For debugging purposes, you could add print statements to the following functions. Position updates could be useful for reacting to a user entering or leaving a certain radius of a store. Status updates help handle situations where the position is no longer available.
override fun onFirstMapLoaded() { mapView.blueDotManager.enable(MPIOptions.BlueDot())}
override fun onBlueDotPositionUpdate(update: MPIBlueDotPositionUpdate) { Log.d("Position", update.position.toString())}
override fun onBlueDotStateChange(stateChange: MPIBlueDotStateChange) { Log.d("Name", stateChange.name.toString()) Log.d("Reason", stateChange.reason.toString())}
Simulating Blue Dot
To display a Blue Dot with the SDK, you'll need the position information in the form of MPICoordinates
. The following is a point in the fictional Mappedin Demo Mall with an accuracy of 2 meter radius on the floor level 0
.
val coords = MPIPosition.MPICoordinates(43.52012478635707, -80.53951744629536, 2.0, 0)
Using our sample coordinate, we can draw the Blue Dot on the map by creating an MPIPosition
and passing it to blueDotManager.updatePosition()
.
override fun onFirstMapLoaded() { mapView.blueDotManager.enable(MPIOptions.BlueDot()) val coords = MPIPosition.MPICoordinates(43.52012478635707, -80.53951744629536, 2.0, 0) val position = MPIPosition(1.0, coords) mapView.blueDotManager.updatePosition(position)}
Blue Dot Display States
- When the Blue Dot position given to the Mappedin Web SDK has a high accuracy (within a few meters) and is on the floor currently displayed, it is displayed as bright blue.
- If the Mappedin Blue Dot is able to detect that a given position update is inaccurate, a semi-transparent blue shadow is displayed underneath the Blue Dot to indicate uncertainty range.
- When the BlueDot is on another floor it will look semi-transparent.
- The uncertainty range is shown also when displaying the Blue Dot on another floor than the currently viewed one.
- 30 seconds after the latest position update, the BlueDot is displayed as greyed out as it's possible that the user has moved but no updates have been received.
Wayfinding From Blue Dot
A common map feature is to provide a user with directions from their current location. The Mappedin Web SDK is able to provide the nearest node to the user’s location, which can be used as the starting point for wayfinding. Pass the user’s destination to the getDirections
method to create a directions object that can be used to draw the path.
override fun onBlueDotPositionUpdate(update: MPIBlueDotPositionUpdate) { val departure = update.nearestNode val destination = mapView.venueData?.locations?.first { it.name == "Destination Name" }
if (departure == null || destination == null) return
mapView.getDirections(to = destination, from = departure) { if (it != null) { mapView.journeyManager.draw(directions = it) } }}
Camera Follow Mode
In some instances, a user may pan the camera away and lose track of their own Blue Dot position. An app may want to snap the camera to the user's location to reorient themselves. While this could also be done using camera controls, an app can also leverage the map STATE to pin the camera to the Blue Dot's location.
By default, the map starts in EXPLORE mode allowing the user to freely control the camera position. To pin the camera to the Blue Dot, set the camera state to FOLLOW with Blue Dot enabled.
mapView.blueDotManager.enable(options = MPIOptions.BlueDot(smoothing = true))mapView.blueDotManager.setState(MPIState.FOLLOW)
The camera will animate to the Blue Dot position whenever it is updated, however, it doesn't zoom or rotate. When providing wayfinding directions, it may be beneficial to orient the camera along the route. Enable this by setting useRotationMode of MPIOptions.BlueDot to true to when calling MPIBlueDotManager.enable().
Rotation mode only works when using the MPIJourneyManager. The camera will not rotate with a path created using MPIPathManager. You can read more about path differences in the A-B Wayfinding guide.
mapView.blueDotManager.enable( options = MPIOptions.BlueDot(smoothing = true, useRotationMode = true))mapView.blueDotManager.setState(MPIState.FOLLOW)
A complete class that uses the code snippets in this guide can be found in the Mappedin Android Github repo: BlueDot.kt