Return to Resources
Integrating Indoor Positioning System with Mappedin SDKs
Feb 15, 2022
4 min read
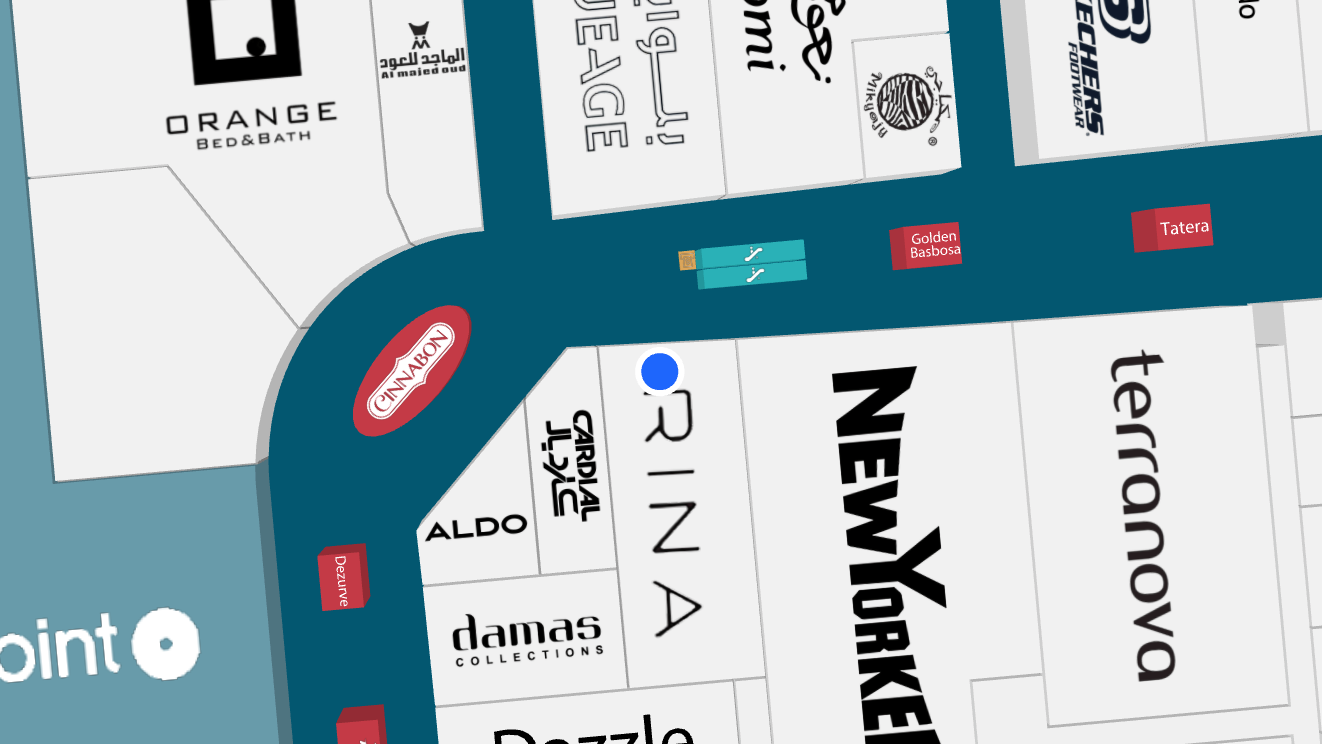
You are here is one of the first things we expect to find on a map today. There is no need to manually triangulate our position based on stars because the device we hold in our hands can already tell us our location. That is if we are outdoors. The story gets more complicated if we move indoors.
With Indoor Positioning Systems (IPS) users can see their precise location represented by a blue dot within the context of an indoor map. Similar to outdoor GPS, users are not required to enter a "start" location and as they begin to follow along a route, the blue dot moves with them, providing an enhanced navigational experience.
Indoor Positioning Systems are valuable components of rich indoor mapping experiences. They ensure that the user does not need to know their location in a venue. This saves both time and confusion, making for a much more pleasant wayfinding experience.
GPS has about 3 meter accuracy when we are outside and have no obstructions such as skyscrapers around us. However, inside a building, GPS is nearly useless. That's why several technologies have been developed to provide indoor positioning in various scenarios such as wayfinding, asset tracking, contact monitoring and smart building applications.
Positioning smarts for indoors
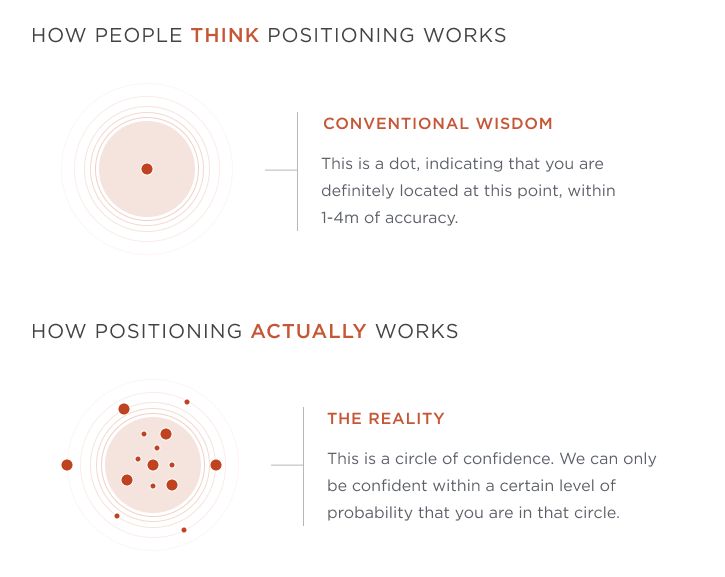
The different indoor positioning systems have different tradeoffs. For example, using beacons requires installing Bluetooth Low Energy (BLE) devices in known positions around the venue. WiFi-based systems use existing infrastructure and requires a radio frequency mapping of the venue. Some systems blend multiple technologies in sensor fusion to further process the positioning data into a more accurate representation of the actual position.
To integrate with the Mappedin SDKs, it does not really matter what underlying technology is used to obtain the indoor position as long as it can provide information about the current floor and the geocoordinates (latitude and longitude). Based on that the Mappedin SDK is able to place the Blue Dot on the map indicating the current position of the device.
{ latitude: 43.51905183293411, longitude: -80.53701846381122, accuracy: 5, // radius in meters floorLevel: 0, // mappedin floor level}
One of the easiest ways to setup Indoor Positioning System is Apple's infrastructure-free positioning combined with Mappedin map rendering and data management.
Integrating positioning data with Mappedin React Native SDKs
Below is the minimal implementation of drawing a map with Mappedin React Native SDK. In 5 minutes, we will build on this code to display the Blue Dot and override the positions from a premade path of geocoordinates. After ensuring everything works as expected, you can easily connect the IPS to provide the position to Mappedin SDK instead
import React from 'react';import { SafeAreaView } from 'react-native';import { MapViewStore, MiMapView, } from '@mappedin/react-native-sdk';
const App = () => { const mapView = React.useRef<MapViewStore>(null);
return ( <SafeAreaView style={{flex: 1}}> <MiMapView style={{flex: 1}} key="mappedin" ref={mapView} options={{ clientId: '<MAPPEDIN_CLIENT_ID>', clientSecret: '<MAPPEDIN_CLIENT_SECRET>', venue: 'mappedin-demo-mall', }} /> </SafeAreaView> );};
export default App;
We have pregenerated a route in positions.json
that can be used for debugging Blue Dot features in mappedin-demo-mall
. Below is the first element of the list, which we will set as our starting coordinate for camera focus. It follows the specification of Geolocation Position of Web APIs.
const location = { "timestamp": 1583957906820, "coords": { "accuracy": 5, "latitude": 43.518976003360244, "longitude": -80.53726260556732, "floorLevel": 0 }, "type": 0}
First, we create a MappedinCoordinate
for our starting location and zoom in the camera to get a closer view of the Blue Dot.
<MiMapView style={{flex: 1}} key="mappedin" ref={mapView} options={{ clientId: '<MAPPEDIN_CLIENT_ID>', clientSecret: '<MAPPEDIN_CLIENT_SECRET>', venue: 'mappedin-demo-mall', }}
onFirstMapLoaded={async () => { const startingCoordinate = mapView.current?.currentMap?.createCoordinate( positions[0].coords.latitude, positions[0].coords.longitude, )!; await mapView.current?.Camera.focusOn({ targets: { coordinates: [startingCoordinate], }, });
}}/>
We set the map state to follow
, which keeps the Blue Dot in the middle of the view. Then we enable the Blue Dot and set the starting location.
// Below our Camera.focusOn mapView.current?.setState(STATE.FOLLOW); mapView.current?.BlueDot.enable(); mapView.current?.overrideLocation(location);
To walk through the list of positions, we can create a simple interval outside of our render
method for overriding the position every few seconds. The position will jump to the start after the route is completed. This code is not optimized and should be replaced with the positioning updates coming from your IPS.
let positionIndex = 0; setInterval(() => { mapView.current?.overrideLocation( positions[positionIndex % positions.length], ); positionIndex++; }, 2000);
Completed sample
Below code sample can be pasted into a fresh React Native starter project with the addition of positions.json
.
import React from 'react';import {SafeAreaView} from 'react-native';
import {MapViewStore, MiMapView, STATE} from '@mappedin/react-native-sdk';import positions from './positions.json';
const App = () => { const mapView = React.useRef<MapViewStore>(null);
let positionIndex = 0; setInterval(() => { mapView.current?.overrideLocation( positions[positionIndex % positions.length], ); positionIndex++; }, 2000);
return ( <SafeAreaView style={{flex: 1}}> <MiMapView style={{flex: 1}} key="mappedin" ref={mapView} options={{ clientId: '<MAPPEDIN_CLIENT_ID>', clientSecret: '<MAPPEDIN_CLIENT_SECRET>', venue: 'mappedin-demo-mall', }} onFirstMapLoaded={async () => { const startingCoordinate = mapView.current?.currentMap?.createCoordinate( positions[0].coords.latitude, positions[0].coords.longitude, )!; await mapView.current?.Camera.focusOn({ targets: { coordinates: [startingCoordinate], }, }); mapView.current?.setState(STATE.FOLLOW); mapView.current?.BlueDot.enable(); mapView.current?.overrideLocation(positions[0]); }} /> </SafeAreaView> );};
export default App;
Summary
Mappedin SDKs are ready to work with any IPS provider that can be integrated with your app to provide geolocation and floor to Mappedin within minutes. The Blue Dot can be enabled and then connected to the position updates in just a few lines of code, delivering accurate indoor positioning and enhanced wayfinding experiences.
If you want to discuss indoor positioning as it relates to your venue, contact us now.